Week 1 - Day 1: Python Basics for Data Science 🚀
- Aakanksha Singh
- Oct 20, 2024
- 8 min read
Objective:
Welcome to the first day of your Data Science, AI, and ML Mastery journey! Today, we’ll focus on the essential Python concepts needed to kick-start your data science career. From setting up your environment to understanding data types, loops, and functions—let’s dive in!
Part 1: Setting Up Your Python Environment
Before coding, it's crucial to set up the right environment for smooth programming. We’ll use Anaconda, which bundles everything you need for scientific computing with Python.
Steps to Install and Use Anaconda:
Download Anaconda: Visit the Anaconda website and download the version for your operating system (Windows, macOS, or Linux).
Install Anaconda: Follow the instructions for your OS.
Launch Anaconda Navigator: Open the dashboard to manage libraries and tools.
Open Jupyter Notebook: Start Jupyter from the Navigator to write and run Python code interactively.
Part 2: Python Basics
Now that we’re all set, let’s learn Python basics! We’ll cover data types, control structures, loops, and functions—the building blocks of any Python program.
1️⃣ Data Types in Python
Python supports various primitive and complex data types:
Primitive Data Types:
Integers (int): Whole numbers, both positive and negative, without a decimal point or fractional part.
Example :
python
Copy code
age = 25
print(type(age)) # Output: <class 'int'>
Floating-point numbers (float): Numbers that have a decimal point, representing real numbers.
Example:
python
Copy code
price = 19.99
print(type(price)) # Output: <class 'float'>
Strings (str): A sequence of characters (letters, numbers, symbols) enclosed in quotes.
Example:
python
Copy code
greeting = "Hello, Data Science!" print(type(greeting)) # Output: <class 'str'>
Booleans (bool): These represent two possible values, True or False.
Example:
python
Copy code
is_raining = False print(type(is_raining)) # Output: <class 'bool'>
Mini-Project 1: Basic Calculator 🧮
Create a simple calculator using integer, float, and string inputs. It should perform addition, subtraction, multiplication, and division based on user input.Steps:
Ask the user to input two numbers.
Ask for the operation (add, subtract, multiply, divide).
Perform the operation and display the result.
Code:
python
Copy code
# Simple Calculator
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
operation = input("Enter the operation (+, -, *, /): ")
if operation == "+":
print("Result:", num1 + num2)
elif operation == "-":
print("Result:", num1 - num2)
elif operation == "*":
print("Result:", num1 * num2)
elif operation == "/":
print("Result:", num1 / num2)
else:
print("Invalid operation")
Complex Data Types:
Lists: Ordered, mutable collections of elements. Lists can store mixed data types (integers, floats, strings).
Example:
python
Copy code
fruits = ["apple", "banana", "cherry"] print(fruits) # Output: ['apple', 'banana', 'cherry']
Tuples: Similar to lists, but immutable (cannot be modified after creation).
Example:
python
Copy code
coordinates = (10, 20) print(coordinates) # Output: (10, 20)
Dictionaries: Unordered collections of key-value pairs, where each key is unique.
Example:
python
Copy code
student = {"name": "Alice", "age": 22, "grade": "A"} print(student) # Output: {'name': 'Alice', 'age': 22, 'grade': 'A'}
Sets: Unordered collections of unique elements.
Example:
python
Copy code
unique_numbers = {1, 2, 3, 3, 4} print(unique_numbers) # Output: {1, 2, 3, 4}
Mini Project 2: Contact Book 📲📠
Create a simple contact book using a dictionary where users can store names and phone numbers. The program should allow adding, viewing, and searching contacts.
Steps:
Create a dictionary to store contacts.
Allow the user to add contacts.
Provide an option to view all contacts or search for a specific one by name.
Code:
python
Copy code
# Contact Book
contacts = {}
while True:
print("1. Add Contact")
print("2. View Contacts")
print("3. Search Contact")
print("4. Exit")
choice = input("Choose an option: ")
if choice == "1":
name = input("Enter name: ")
phone = input("Enter phone number: ")
contacts[name] = phone
print("Contact added.")
elif choice == "2":
print("Contacts:")
for name, phone in contacts.items():
print(f"{name}: {phone}")
elif choice == "3":
search_name = input("Enter the name to search: ")
if search_name in contacts:
print(f"{search_name}: {contacts[search_name]}")
else:
print("Contact not found.")
elif choice == "4":
break
else:
print("Invalid option.")
Practice Set 1:
Create a list that stores your favorite fruits.
Create a dictionary that stores your name, age, and favorite hobby.
Print the type of a float and an integer.
Resources:
W3Schools: Python Data Types: https://www.w3schools.com/python/python_datatypes.asp
Official Python Documentation: https://docs.python.org/3/
Set Up Your Environment:
Download and Install Anaconda: Anaconda is a popular distribution for Python, which includes many libraries for data science. Visit the Anaconda website and download the installer for your operating system. Follow the installation instructions.
Launch Jupyter Notebook: Anaconda Navigator comes with Jupyter Notebook, a web application for writing and running Python code interactively. Open Anaconda Navigator and click on "Launch" under Jupyter Notebook.
Create a New Python File:In Jupyter, create a new notebook by selecting "New" > "Python 3" from the top-right menu. Name it data_types.ipynb.
Write and Execute Code:In the first cell of your Jupyter Notebook, enter the following code:
python
Copy code
# Example of different data types
integer_var = 10
float_var = 20.5
string_var = "Hello, Python!"
boolean_var = True
print(type(integer_var)) # Output: <class 'int'>
print(type(float_var)) # Output: <class 'float'>
print(type(string_var)) # Output: <class 'str'>
print(type(boolean_var)) # Output: <class 'bool'>
Run the cell by clicking the "Run" button or pressing Shift + Enter. Observe the output below the cell.
2️⃣Control Structures: Making Decisions in Code
Control structures allow us to control the flow of the program based on conditions or repetitions. Control structures are essential for decision-making in your code. They allow your program to choose different paths of execution based on conditions.
Conditional Statements:These allow us to execute different blocks of code based on conditions.
If Statement: Executes a block of code if a specified condition is True.
Example:
python
Copy code
age = 18
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
Elif and Else:
Elif Statement: Short for "else if," it allows you to check multiple conditions sequentially.
Example:
python
Copy code
age = 70
if age < 18:
print("You are a minor.")
elif age < 65:
print("You are an adult.")
else:
print("You are a senior.")
Else Statement: Executes when all preceding conditions are False.
Example:
python
Copy code
score = 85
if score >= 90:
print("Grade: A")
elif score >= 80:
print("Grade: B")
elif score >= 70:
print("Grade: C")
else:
print("Grade: D")
3️⃣Loops: Automating Repetitive Tasks
Loops allow you to execute a block of code repeatedly.
For Loop: Iterates over a sequence (list, tuple, string) or range.
Example:
python
Copy code
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
While Loop: Repeats as long as a condition is True.
Example:
python
Copy code
count = 0
while count < 5:
print("Count:", count)
count += 1
Mini-Project 3: Number Guessing Game 🎲
Build a simple number guessing game where the computer randomly selects a number, and the user has to guess it.Steps:
Import the random module to generate random numbers.
Define a range for the random number.
Prompt the user to guess the number.
Provide hints if the guess is too high or too low.
Code:
python
Copy code
# Number Guessing Game
import random
number_to_guess = random.randint(1, 100)
guesses_taken = 0
print("Guess a number between 1 and 100!")
while guesses_taken < 10:
guess = int(input("Take a guess: "))
guesses_taken += 1
if guess < number_to_guess:
print("Your guess is too low.")
elif guess > number_to_guess:
print("Your guess is too high.")
else:
break # This condition is the correct guess!
if guess == number_to_guess:
print(f"Good job! You guessed the number in {guesses_taken} tries!")
else:
print(f"Sorry, the number was {number_to_guess}.")
Practice Set 2:
Write a program that asks the user for a number and determines if it is even or odd.
Create a loop that prints the first 10 squares (1, 4, 9, 16, ...).
Write a program that prints the multiplication table for a number given by the user.
Resources:
Real Python: Control Flow in Python: https://realpython.com/python-conditional-statements/
Official Python Documentation: https://docs.python.org/3/
Tasks:
Create a New Python File:In Jupyter, create a new notebook by selecting "New" > "Python 3" from the top-right menu. Name it control_structures.ipynb.
Write and Execute Code:In the first cell of your Jupyter Notebook, enter the following code:
python
Copy code
# Example of control structures
number = 7
if number % 2 == 0:
print(f"{number} is even.")
else:
print(f"{number} is odd.")
# For loop example
for i in range(1, 6):
print(i * i)
Run the cell and check the output.
4️⃣Functions: Reusable Code Blocks
Functions are reusable blocks of code that perform a specific task. They help organize code, avoid repetition, and enhance readability.
Defining a Function:
You can define a function using the def keyword followed by the function name and parentheses.
Example:
python
Copy code
def greet(name):
return f"Hello, {name}!"
print(greet("Alice")) # Output: Hello, Alice!
Function Parameters:
You can pass parameters to functions, allowing them to operate on input data.
Example:
python
Copy code
def add(a, b):
return a + b
result = add(5, 10)
print(result) # Output: 15
Mini Project 4: Temperature Converter🧪⚗
Build a temperature converter that converts Celsius to Fahrenheit and vice versa using functions.
Steps:
Create two functions: one for converting Celsius to Fahrenheit and another for Fahrenheit to Celsius.
Prompt the user for input and perform the conversion.
Code:
python
Copy code
# Temperature Converter
def celsius_to_fahrenheit(celsius):
return (celsius * 9/5) + 32
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
temperature = float(input("Enter temperature: "))
unit = input("Is this Celsius or Fahrenheit? (C/F): ").upper()
if unit == "C":
converted = celsius_to_fahrenheit(temperature)
print(f"{temperature}°C is {converted}°F")
elif unit == "F":
converted = fahrenheit_to_celsius(temperature)
print(f"{temperature}°F is {converted}°C")
else:
print("Invalid unit")
Practice Set 3:
Create a function that checks if a number is prime.
Write a function that calculates the factorial of a given number.
Define a function that takes a list of numbers and returns the average.
Resources:
GeeksforGeeks: Python Functions
Tasks and Practice Sets for Today
📋 Practice Tasks:
Data Types:
Create a list of your favorite fruits and print each fruit using a loop.
Create a dictionary with your name, age, and favorite hobby. Print the dictionary.
Loops:
Write a program to print numbers from 1 to 10 using a for loop.
Functions:
Write a function that takes two numbers and returns their sum.
Homework Assignment
To reinforce your learning, complete the following tasks:
Data Types Practice:
Create a new notebook named practice_data_types.ipynb.
Define a variable for each data type discussed and print their types.
Create a list of at least five different fruit names and print them using a loop.
Control Structures Practice:
Write a program that checks whether a number is positive, negative, or zero using conditional statements.
Include error handling to ensure the user inputs a valid number.
Loops Practice:
Create a notebook called fibonacci_sequence.ipynb.
Write a program to print the Fibonacci sequence up to the 10th number using a loop.
Function Practice:
Create a new function that takes two numbers as parameters and returns their average.
Call this function with different pairs of numbers and print the results.
Conclusion:
You have learned fundamental concepts of Python programming, including data types, control structures, loops, and functions. You practiced through hands-on examples, reinforcing your understanding.
Reflection:
Take a moment to reflect on what you learned today. Write down three things you found interesting or challenging in your notebook. This will help solidify your understanding and identify areas for further study.
Next Steps:
Review the material covered today and complete any tasks you might have missed.
Prepare for Day 2, which will focus on data structures like lists, tuples, sets, and dictionaries in detail, along with practice exercises.
Resources for Further Learning
Books:
"Automate the Boring Stuff with Python" by Al Sweigart.
"Python Crash Course" by Eric Matthes.
Online Courses:
Websites:
GeeksforGeeks
Kaggle
That’s it for Day 1!
Great job getting through the first day! 🎉 Keep practicing, and don’t forget to reflect on what you’ve learned. 😊
If you’d like more practice sets, assignments, or project tasks, just let me know, and I’ll share a PDF with additional resources.
Let’s collaborate and learn together—feel free to comment if you run into any issues or need help. See you tomorrow for Day 2! 🚀
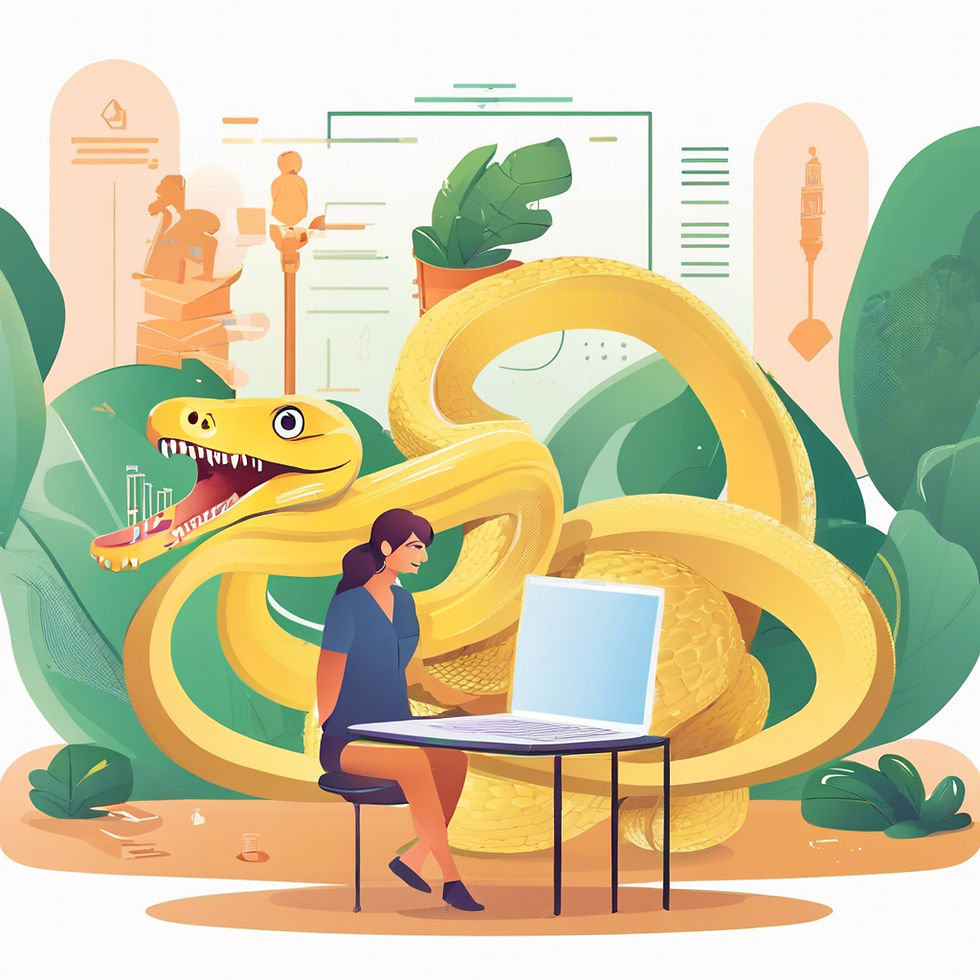
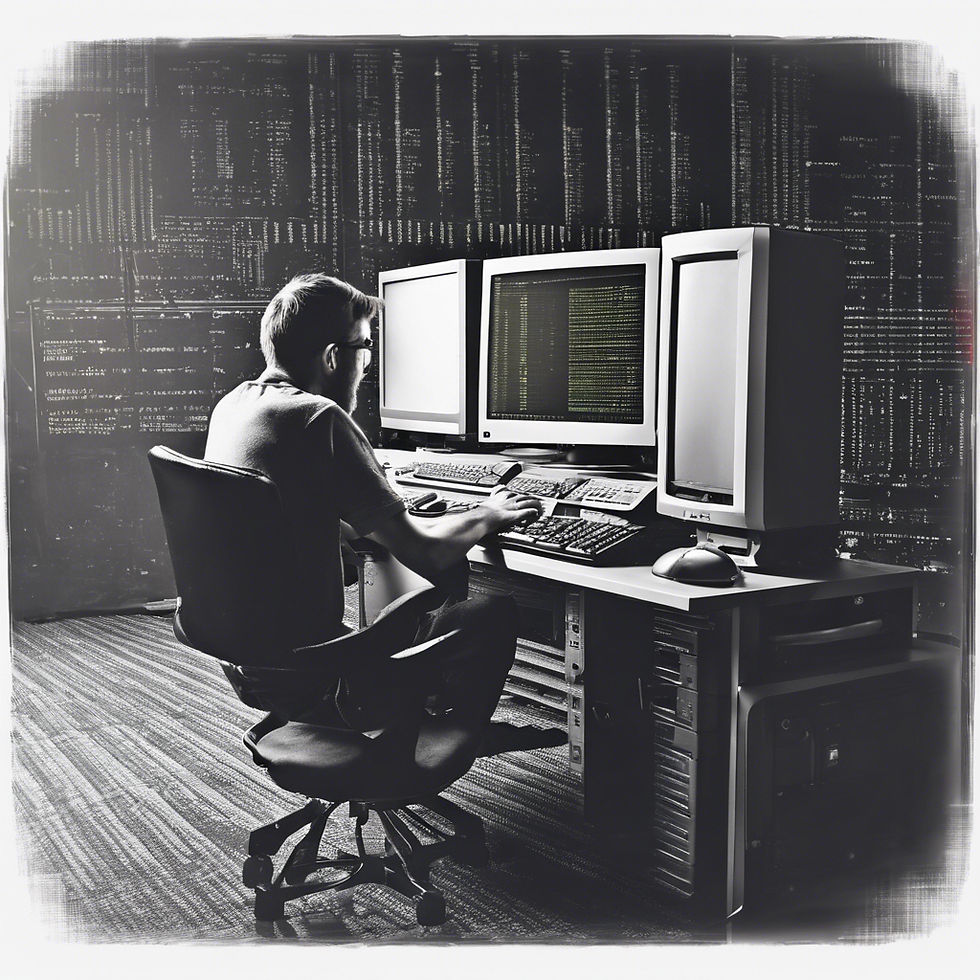
Comments